Test
[Jest] Jest 확장 프로그램 Jest-extended 사용하기
jalalja
2024. 12. 15. 01:31
Jest-extended란?
더보기
- Jest-extended란?
- jest-extended는 Jest에서 사용할 수 있는 추가적인 matcher들을 제공하는 확장 라이브러리입니다.
- Jest에서 기본적으로 제공하는 matcher(toBe, toEqual, toContain 등) 외에도 다양한 상황에서 유용한 matcher들을 추가로 사용할 수 있게 해줍니다.
- jest-extended를 사용하면 테스트의 가독성과 표현력을 높일 수 있습니다.
Jest-extended 설치 (TypeScript 사용)
더보기
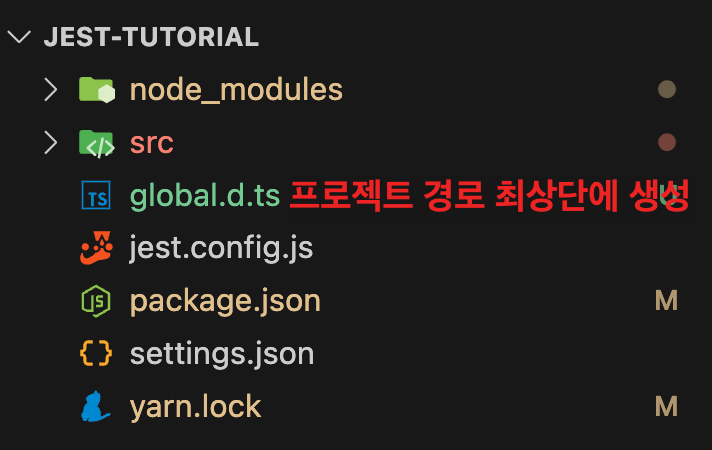
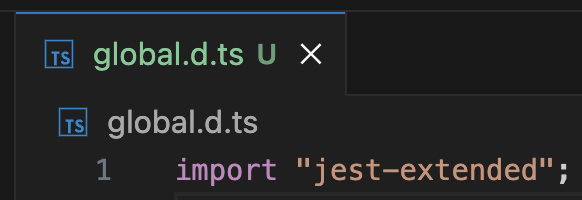
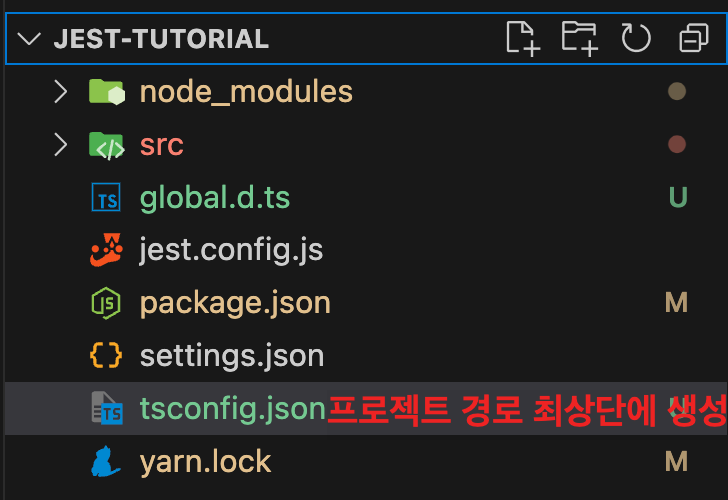
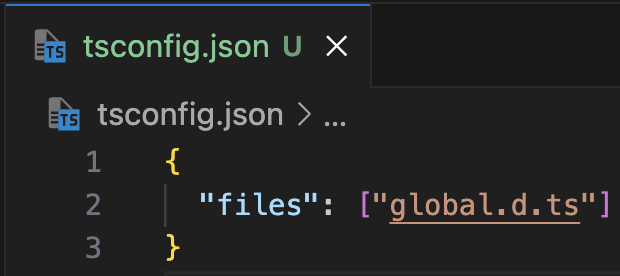
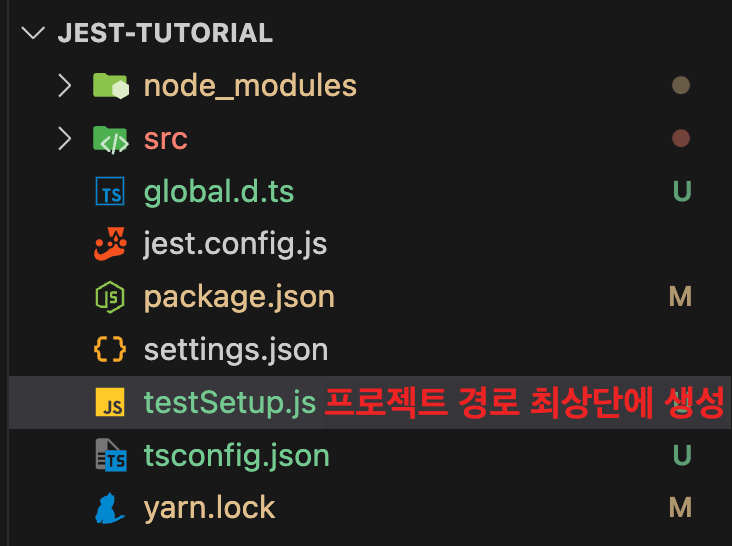
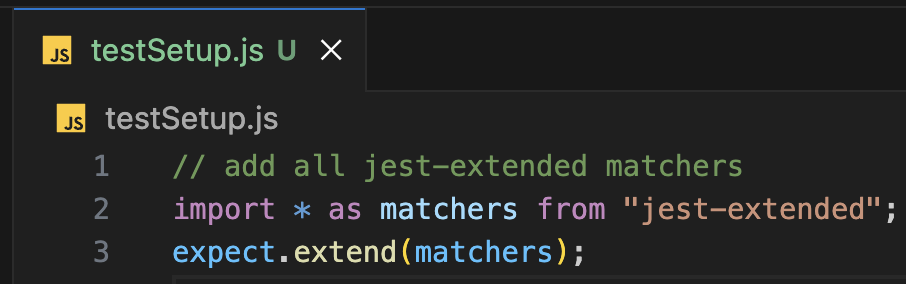
- jest-extended 설치
yarn add -D jest-extended
- global.d.ts 파일 생성
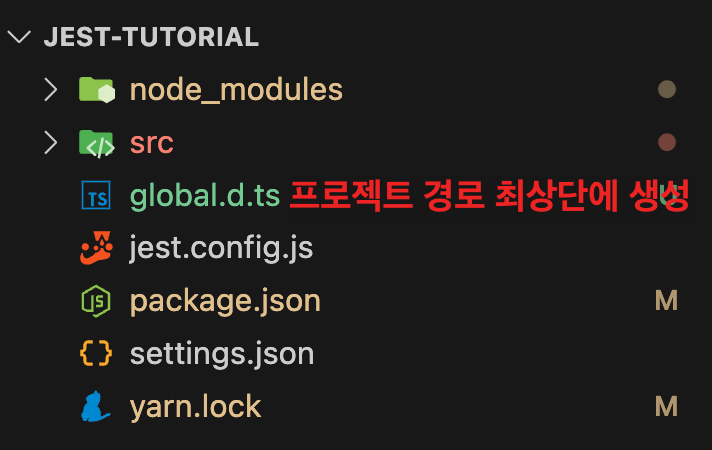
- global.d.ts 파일 설정
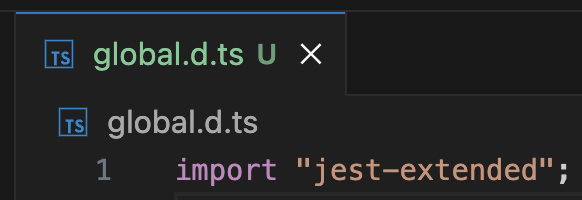
import 'jest-extended';
- tsconfig.json 파일 생성
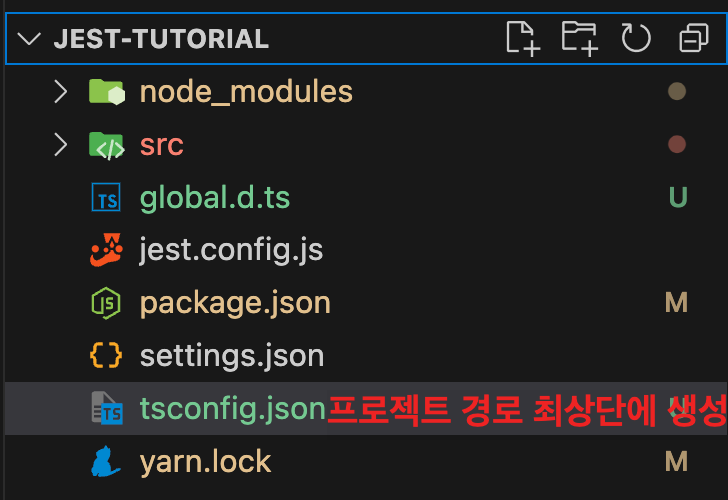
- tsconfig.json 파일 설정
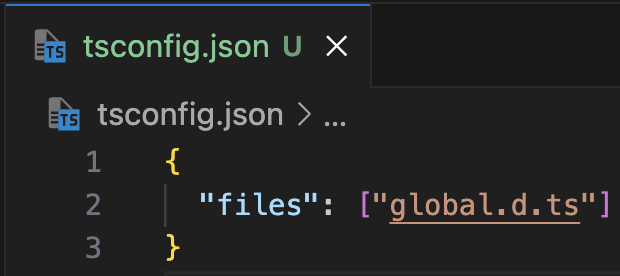
{
"files": ["global.d.ts"]
}
- testSetup.js 파일 생성
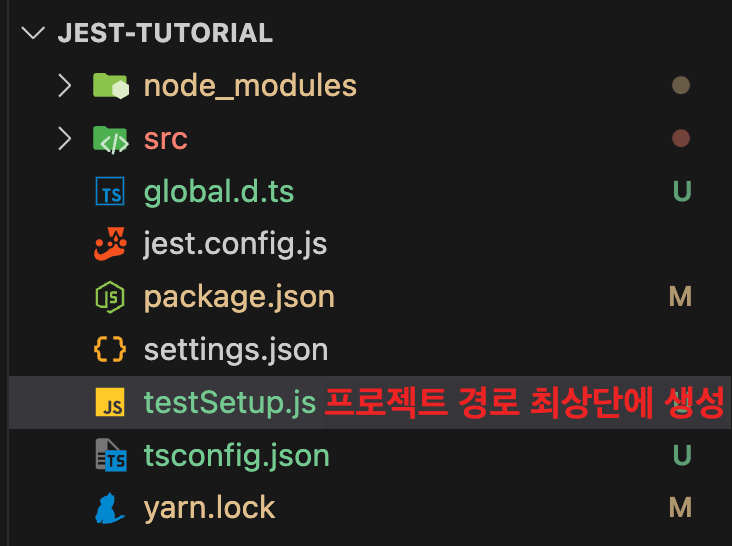
- testSetup.js 파일 설정
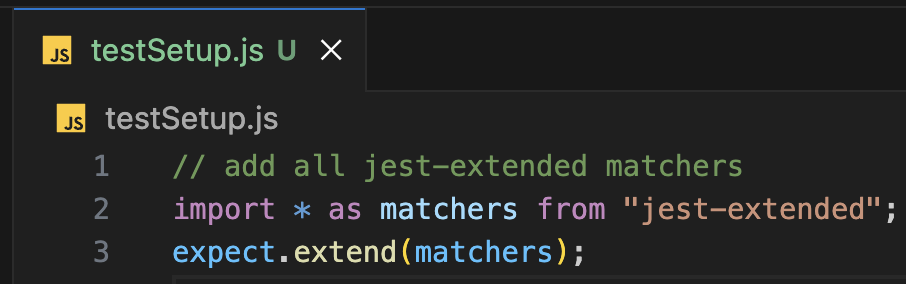
// add all jest-extended matchers
import * as matchers from "jest-extended";
expect.extend(matchers);
- jest.config.js 파일 설정
/** @type {import('ts-jest').JestConfigWithTsJest} **/
export default {
testEnvironment: "node",
transform: {
"^.+.tsx?$": ["ts-jest", {}],
},
testRegex: "(/__tests__/.*|(\\.|/)(test|spec))\\.[jt]sx?$",
// 추가
setupFilesAfterEnv: ["./testSetup.js"]
};
jest-extended 설정 완료 후 생긴 에러
더보기

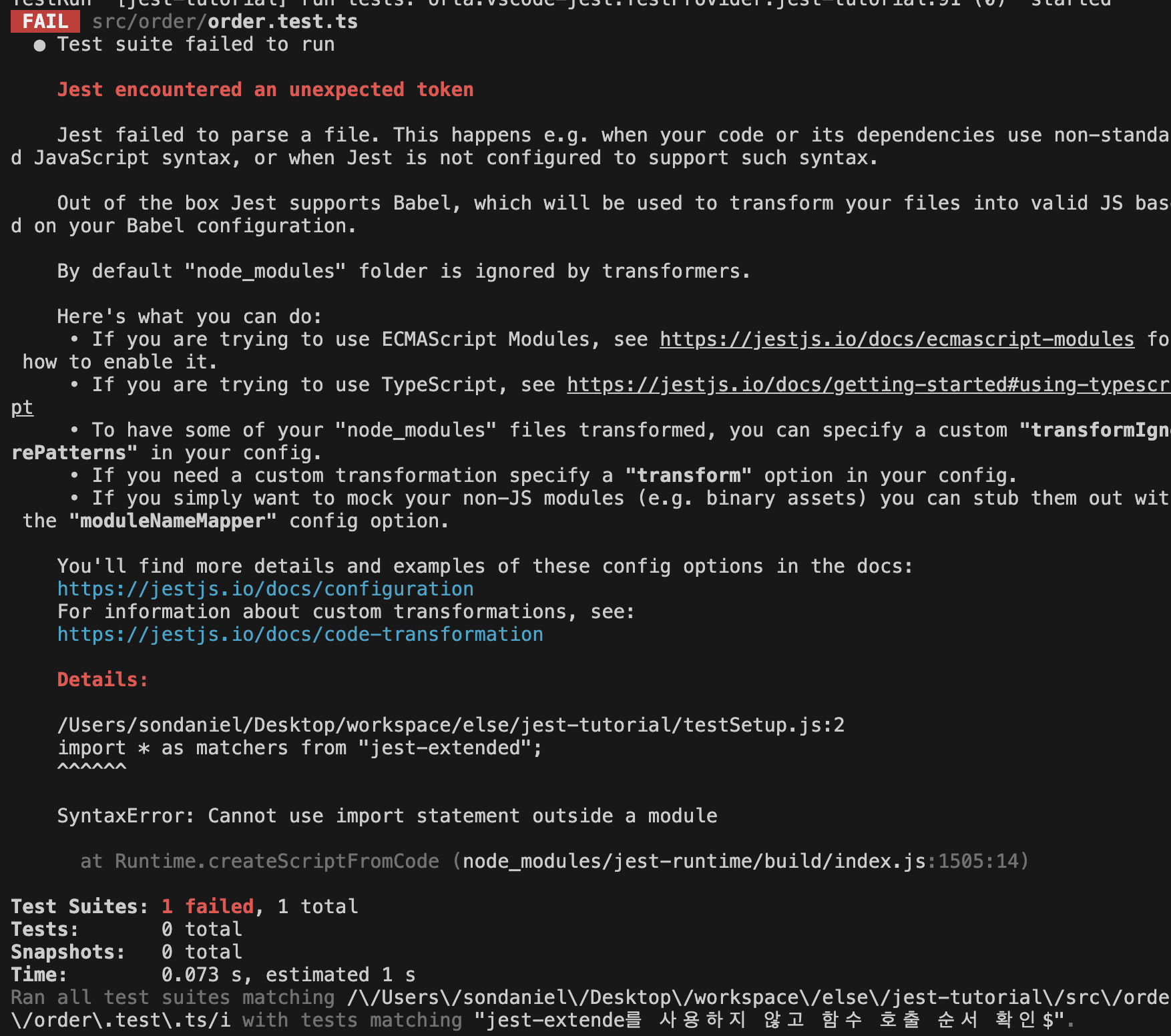
- VS Code에서 지원하는 Jest extension을 사용한 테스트 버튼 클릭으로 테스트 실행 시 에러 발생
- import는 ECMAScript 모듈이기 때문에 jest에서 사용하려면 추가적인 설정이 필요하다는 에러

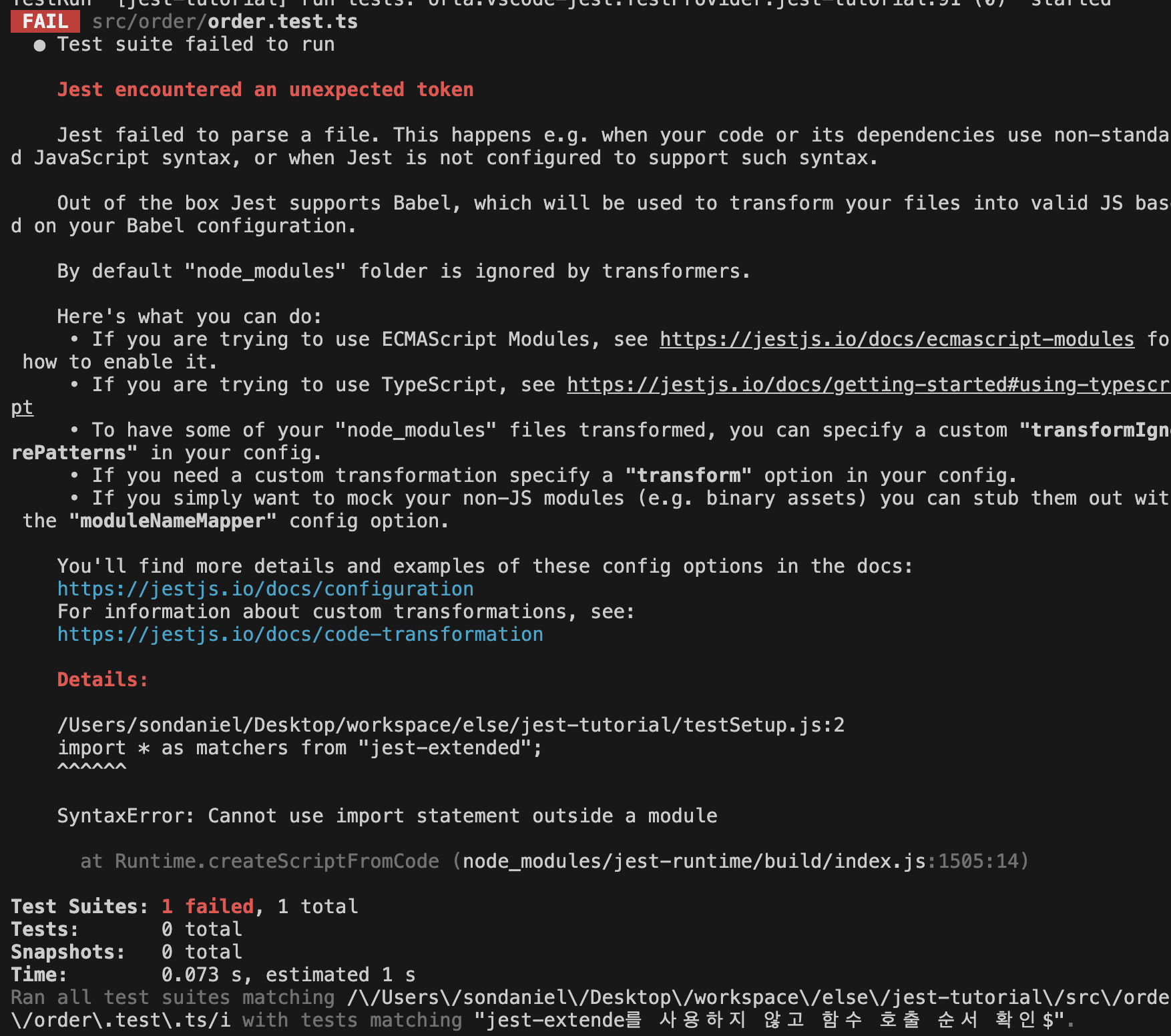
- 해결1. (JavaScript를 사용하는 경우)
- 하단의 블로그 -> 기본 사용법 (Babel X) 참고
[Jest] 개념 & 기본 사용법 정리
Jest 란?- Jest는 Facebook에서 개발한 JavaScript 테스트 프레임워크로, React를 포함한 다양한 JavaScript 애플리케이션의 유닛 테스트와 통합 테스트를 쉽게 작성하고 실행할 수 있도록 도와줍니다.Jest 설
jalalja.tistory.com
- 해결2. (TypeScript를 사용하는 경우)
- testSetup.js 파일 -> testSetup.ts로 변경
- jest.config.js 파일 -> setupFilesAfterEnv: ["./testSetup.ts"]로 수정
- 공통 해결3. (JavaScript, TypeScript 모두 가능)
- testSetup.js 파일 -> const matchers = require("jest-extended");로 import 구문 변경
Jest-extended 사용
더보기
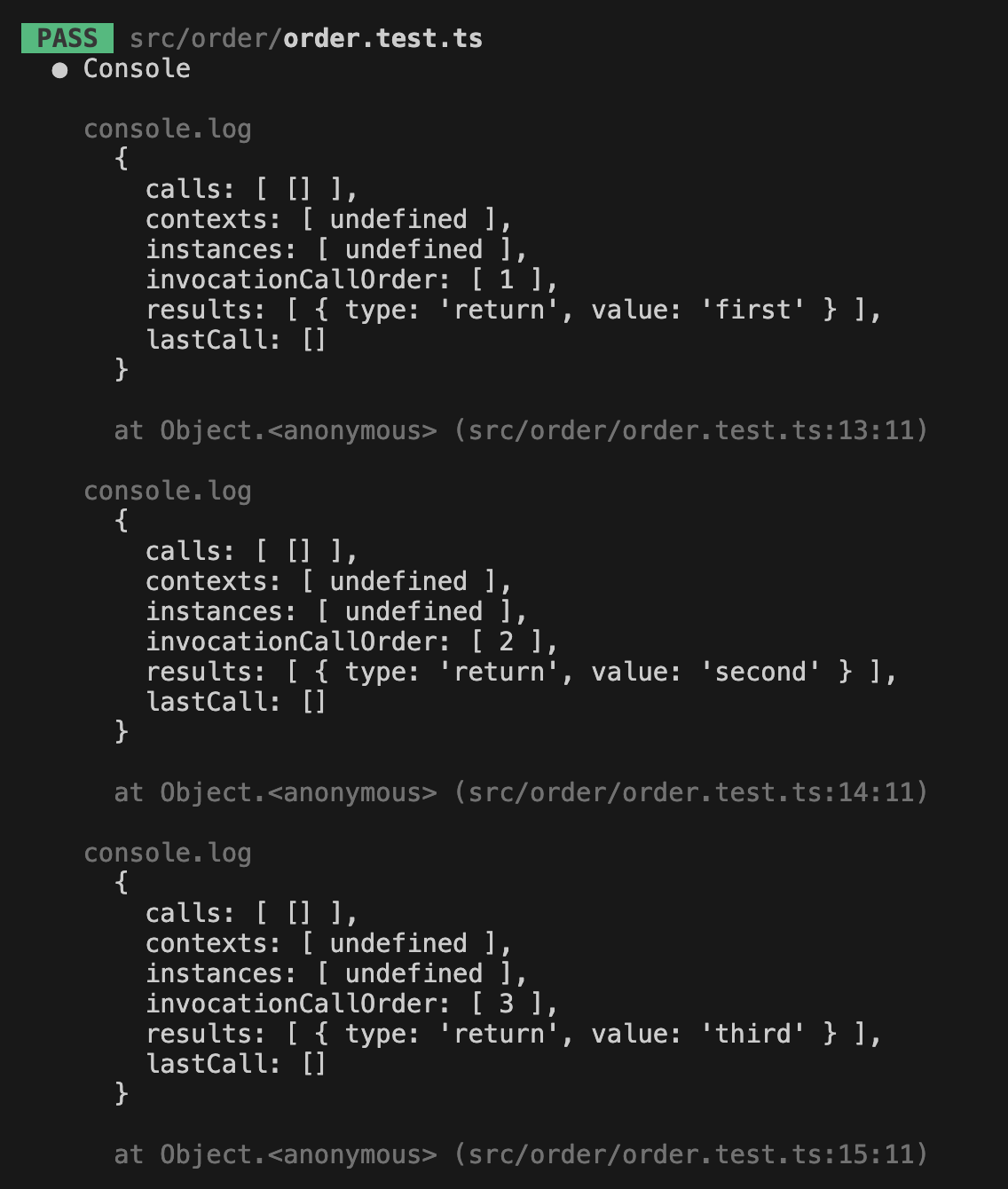
- jest-extended를 사용하지 않고 함수의 호출 순서를 확인하는 코드
import { first, second, third } from "./order";
// jest-extende를 사용하지 않고 함수 호출 순서 확인
test("jest-extende를 사용하지 않고 함수 호출 순서 확인", () => {
const spy1 = jest.fn(first);
const spy2 = jest.fn(second);
const spy3 = jest.fn(third);
spy1();
spy2();
spy3();
console.log(spy1.mock);
console.log(spy2.mock);
console.log(spy3.mock);
expect(spy1.mock.invocationCallOrder[0]).toBeLessThan(
spy2.mock.invocationCallOrder[0]
);
expect(spy3.mock.invocationCallOrder[0]).toBeGreaterThan(
spy2.mock.invocationCallOrder[0]
);
});
- spy1.mock에 담긴 정보를 사용해 비교
- mock 안에 있는 invocationCallOrder를 사용해 호출된 순서 확인 가능
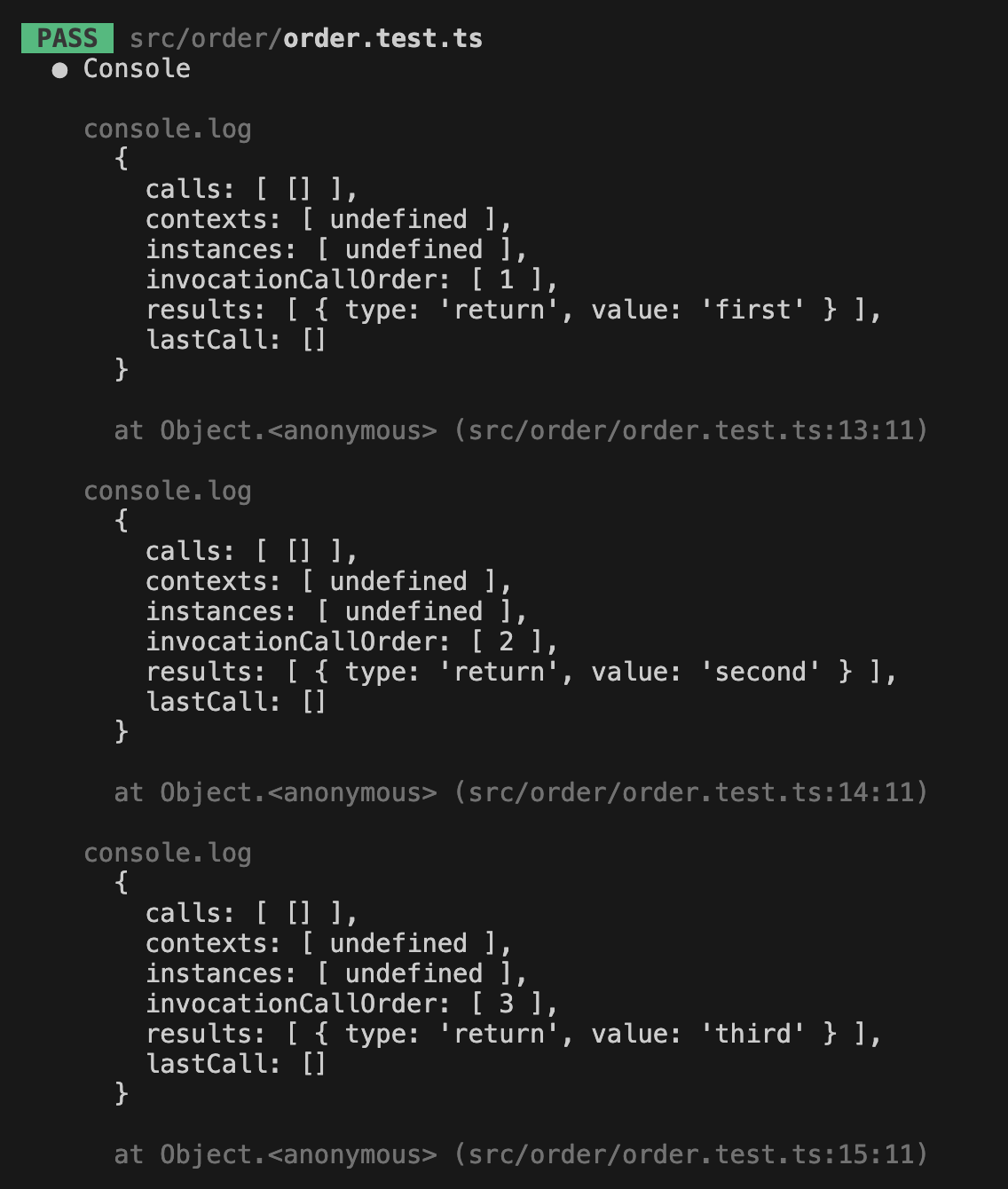
- 직접 mock 데이터에 접근해야하는 번거로움 + 가독성이 떨어지는 문제가 발생하여 jest-extended를 사용한 방식으로 대체
import { first, second, third } from "./order";
// jest-extende를 사용해 함수 호출 순서 확인
test("jest-extende를 사용해 함수 호출 순서 확인", () => {
const spy1 = jest.fn(first);
const spy2 = jest.fn(second);
const spy3 = jest.fn(third);
spy1();
spy2();
spy3();
// expect(spy1.mock.invocationCallOrder[0]).toBeLessThan(
// spy2.mock.invocationCallOrder[0]
// );
expect(spy1).toHaveBeenCalledBefore(spy2);
// expect(spy3.mock.invocationCallOrder[0]).toBeGreaterThan(
// spy2.mock.invocationCallOrder[0]
// );
expect(spy3).toHaveBeenCalledAfter(spy2);
});
다양한 matcher참고
Matchers | jest-extended
- .pass(message)
jest-extended.jestcommunity.dev